I'm trying to get started with evernote SDK, i'm using ubuntu 13.04. I installed the SDK via: pip install evernote but when i want to test it using: python -c 'from evernote.api.client import EvernoteClient' i got this: Traceback (most recent call last): File ', line 1, in ImportError: No module named api. Evernote SDK for Python 3. Evernote API version 1.25. This is a test SDK! The official Evernote SDK for Python doesn't support Python 3 yet; this repository is an experiment as we try to migrate. This SDK contains wrapper code used to call the Evernote Cloud API from Python. The SDK also contains a. It was surprisingly easy: the script is less than 150 lines of python. EverNote team had the good sense of using sqlite for their database storage. A little bit of reverse-engineering of the database file with SQLite Manager revealed that the author liked the letter Z and that the schema is straightforward. Unfortunately the Python API isn't up-to-date with that, but it looks like it would just need to be automatically generated from the Thrift definitions. I'll poke around and see if I can make that happen. Meanwhile I see you've already done a little patching of the client yourself, so you could try to making the Python UserStore function for it. Evernote Python SDK by Evernote: The Evernote Python SDK by Evernote is the official Python implementation by Evernote. This SDK calls the Evernote Cloud API which provides data collection services. Developers need to obtain an API key.
How to install evernote
- Download and install ActivePython
- Open Command Prompt
- Type
pypm install evernote
Python 2.7 | Python 3.2 | Python 3.3 | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Windows (32-bit) |
| |||||||||||||||
Windows (64-bit) |
| |||||||||||||||
Mac OS X (10.5+) |
| |||||||||||||||
Linux (32-bit) |
| |||||||||||||||
Linux (64-bit) |
|
Links
Author
License
Dependencies
Depended by
Imports
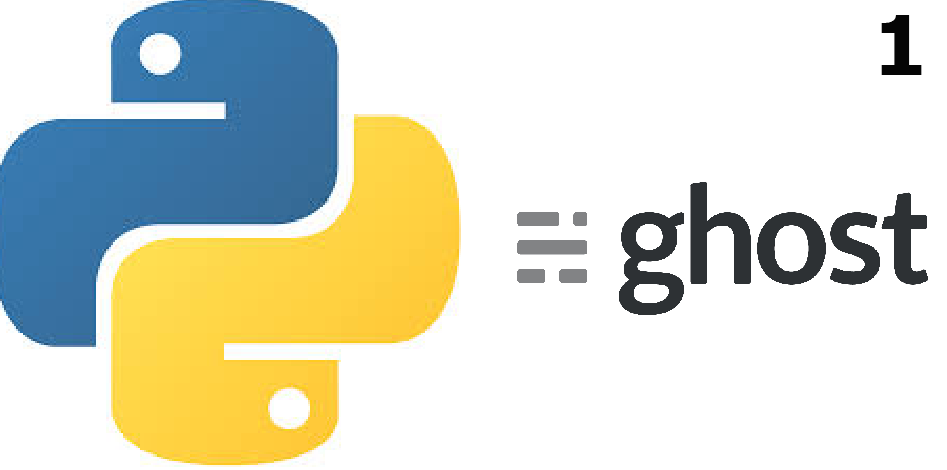
Lastest release
Evernote API version 1.25
Overview
This SDK contains wrapper code used to call the Evernote Cloud API from Python.
The SDK also contains a sample script. The code demonstrates the basic use of the SDK for single-user scripts. Real web applications must use OAuth to authenticate to the Evernote service.

Prerequisites
Evernote Python Api Examples
In order to use the code in this SDK, you need to obtain an API key from http://dev.evernote.com/documentation/cloud. You'll also find full API documentation on that page.
In order to run the sample code, you need a user account on the sandbox service where you will do your development. Sign up for an account at https://sandbox.evernote.com/Registration.action
Evernote Python Api Download
In order to run the client client sample code, you need a developer token. Get one at https://sandbox.evernote.com/api/DeveloperToken.action
Getting Started - Client
The code in sample/client/EDAMTest.py demonstrates the basics of using the Evernote API, using developer tokens to simplify the authentication process while you're learning.
Open sample/client/EDAMTest.py
Scroll down and fill in your Evernote developer token.
On the command line, run the following command to execute the script:
`bash$ export PYTHONPATH=../../lib; python EDAMTest.py`
Getting Started - Django with OAuth
Web applications must use OAuth to authenticate to the Evernote service. The code in sample/django contains a simple web apps that demonstrate the OAuth authentication process. The application use the Django framework. You don't need to use Django for your application, but you'll need it to run the sample code.
Install django, oauth2 and evernote library. You can also use requirements.txt for pip.
Open the file oauth/views.py
Fill in your Evernote API consumer key and secret.
On the command line, run the following command to start the sample app:
`bash$ python manage.py runserver`
Open the sample app in your browser: http://localhost:8000
Getting Started - Pyramid with OAuth
If you want to use Evernote API with Pyramid, the code in sample/pyramid will be good start.
Install the sample project using pip on your command line like this.
`bash$ pip install -e .`
Open the file development.ini
Fill in your Evernote API consumer key and secret.
On the command line, run the following command to start the sample app:
`bash$ pserve development.ini`
Open the sample app in your browser: http://localhost:6543
Usage
### OAuth ###```pythonclient = EvernoteClient(
System Message: WARNING/2 (<string>, line 70); backlink
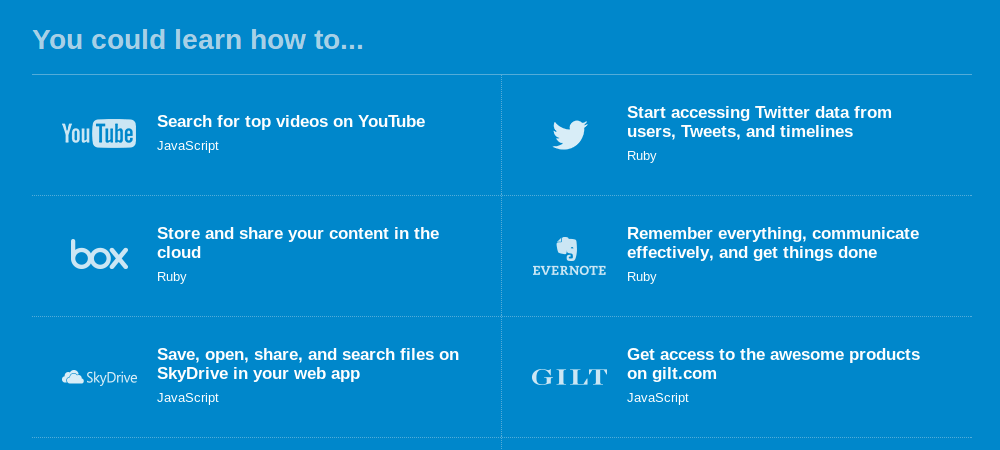
Evernote Python Api Tutorial
Inline literal start-string without end-string.System Message: WARNING/2 (<string>, line 70); backlink
Inline interpreted text or phrase reference start-string without end-string.System Message: ERROR/3 (<string>, line 73)
Unexpected indentation.consumer_key='YOUR CONSUMER KEY',consumer_secret='YOUR CONSUMER SECRET',sandbox=True # Default: True
System Message: WARNING/2 (<string>, line 76)
Block quote ends without a blank line; unexpected unindent.)request_token = client.get_request_token('YOUR CALLBACK URL')client.get_authorize_url(request_token)
System Message: ERROR/3 (<string>, line 79)
Unexpected indentation.
=> https://sandbox.evernote.com/OAuth.action?oauth_token=OAUTH_TOKEN
System Message: WARNING/2 (<string>, line 80)
Block quote ends without a blank line; unexpected unindent.```To obtain the access token```pythonaccess_token = client.get_access_token(
System Message: WARNING/2 (<string>, line 80); backlink
Inline literal start-string without end-string.System Message: WARNING/2 (<string>, line 80); backlink
Inline literal start-string without end-string.System Message: WARNING/2 (<string>, line 80); backlink
Inline interpreted text or phrase reference start-string without end-string.System Message: ERROR/3 (<string>, line 84)
Unexpected indentation.request_token['oauth_token'],request_token['oauth_token_secret'],request.GET.get('oauth_verifier', ')
System Message: WARNING/2 (<string>, line 87)

)
Now you can make other API calls`pythonclient = EvernoteClient(token=access_token)note_store = client.get_note_store()notebooks = note_store.listNotebooks()`
### UserStore ###Once you acquire token, you can use UserStore. For example, if you want to call UserStore.getUser:`pythonclient = EvernoteClient(token=access_token)user_store = client.get_user_store()user_store.getUser()`You can omit authenticationToken in the arguments of UserStore functions.
### NoteStore ###If you want to call NoteStore.listNotebooks:`pythonnote_store = client.get_note_store()note_store.listNotebooks()`
### NoteStore for linked notebooks ###If you want to get tags for linked notebooks:`pythonlinked_notebook = note_store.listLinkedNotebooks()[0]shared_note_store = client.getSharedNoteStore(linked_notebook)shared_notebook = shared_note_store.getSharedNotebookByAuth()shared_note_store.listTagsByNotebook(shared_notebook.notebookGuid)`
### NoteStore for Business ###If you want to get the list of notebooks in your business account:`pythonbusiness_note_store = client.get_business_note_store()business_note_store.listNotebooks()`
### References ###- Evernote Developers: http://dev.evernote.com/- API Document: http://dev.evernote.com/documentation/reference/
